MediatR
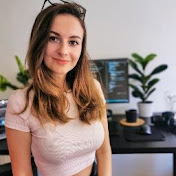
Are you tired of tangled code and complex communication between components? Look no further. MediatR, a lightweight mediator library in .NET, is here to simplify your life. By following the mediator pattern, it enables loose coupling and better organization of your codebase. With MediatR, you can decouple request handling from the rest of your application, promoting separation of concerns like never before.
Gone are the days of struggling with tightly coupled dependencies in software architecture. MediatR empowers developers to create cleaner and more maintainable applications by providing a mediator implementation. Say goodbye to endless lines of code for inter-component communication in the user interface and embrace a simpler approach that boosts productivity. So why wait? Let's dive into the world of MediatR and unlock a new level of efficiency in your program development workflow.
Get ready to update your core web application with MediatR - the game-changer that simplifies communication between components while promoting the separation of concerns. Join us and revolutionize how you build software.
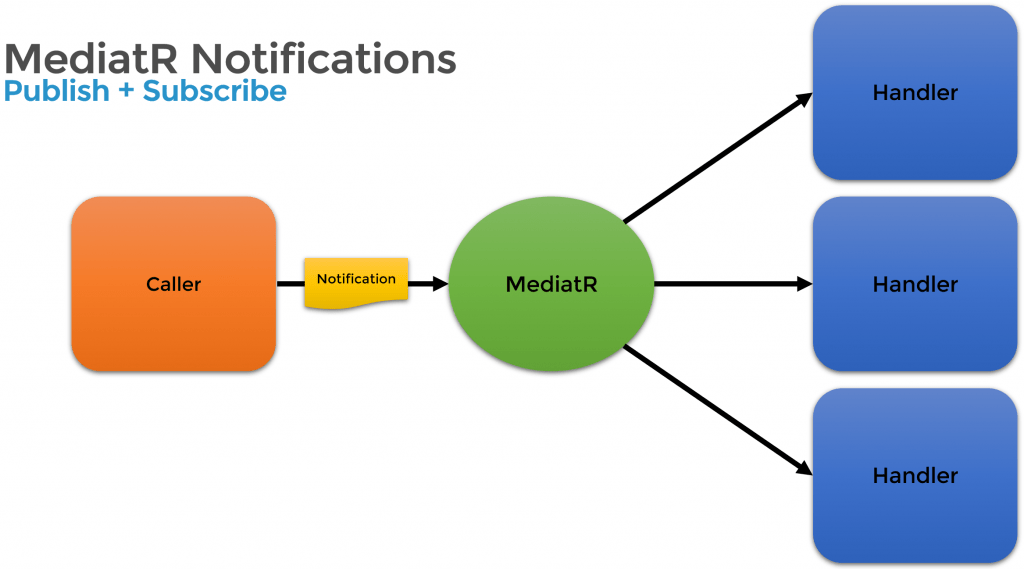
The Purpose and Benefits of MediatR
MediatR is a powerful library that offers numerous benefits to web application developers, making it an essential tool in modern software development. Let's explore some of the key advantages of using MediatR in a public class or interface article.
Achieving Cleaner Code
One of the primary benefits of incorporating MediatR into your project is its ability to help achieve cleaner code. By reducing dependencies and improving maintainability, MediatR simplifies the overall codebase. With this simple public class library, you can eliminate tight coupling between components by decoupling the sender and receiver of requests, thus improving the organization and record of models.
By leveraging the simple mediator pattern, MediatR allows you to send action messages between different parts of your application without them being explicitly aware of each other. This loose coupling promotes modularization and enhances code readability. As a result, maintaining and extending your codebase becomes significantly easier, especially when dealing with public class content.
Implementing CQRS Pattern
MediatR seamlessly enables the implementation of Command Query Responsibility Segregation (CQRS) pattern in ASP.NET Core or .NET 6 applications. CQRS separates read operations (queries) from write operations (commands), providing a clear distinction between data retrieval and modification. With MediatR, developers can easily create a public class to record and order the content for CQRS implementation in their applications.
With MediatR's support for CQRS, you can easily handle commands, queries, and notifications in a centralized manner using the public class. This approach fosters better scalability as you can optimize read-heavy or write-heavy scenarios independently. By segregating concerns based on intent, you can design more efficient systems that align with specific business requirements for the application request. Additionally, the FakeDataStore can be utilized to simulate data storage for testing purposes.
Centralized Message Handling
MediatR promotes a centralized approach to message handling within your application by using the public class. Instead of scattering command or query logic across various controllers or services, MediatR allows you to define handlers for each type of message in one central location, such as the FakeDataStore . This approach simplifies the order of handling messages and makes it easier to manage and maintain your application. So, if you wanna streamline your message handling process, MediatR is the way to go.
This centralized message handling using the MediatR library simplifies maintenance efforts by consolidating related functionality into dedicated handler classes. It also enhances reusability as these handlers can be easily shared among different parts of your application, making it easier to order and organize your code.
For example, when an order is received, MediatR automatically routes it to the appropriate handler based on its type. Similarly, queries can be processed by their respective handlers, ensuring consistent and efficient message handling throughout your application. Join us to experience this seamless order management system.
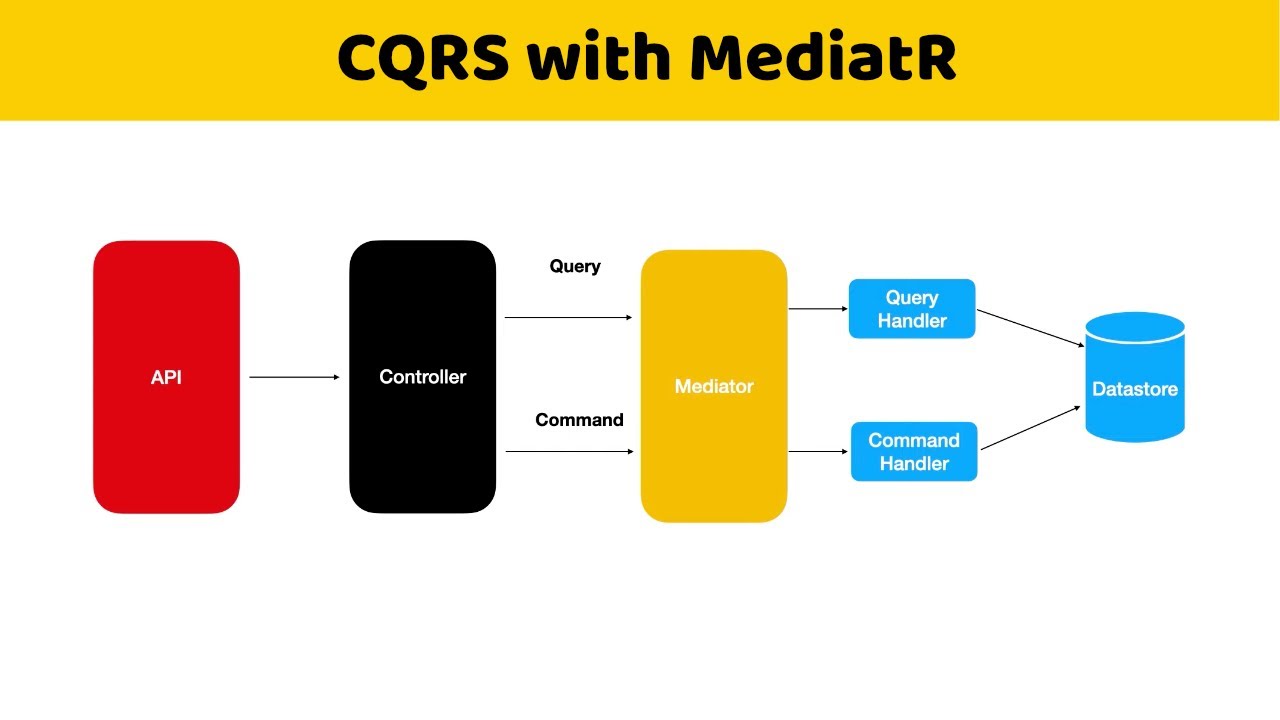
Implementing CQRS and MediatR in ASP.NET Core or .NET6
To achieve a more maintainable and scalable codebase, developers can join us in adopting the Command Query Responsibility Segregation (CQRS) architectural pattern. By combining CQRS with MediatR, clear separation of read and write operations within an application is possible. This segregation emphasizes the order of commands that modify data from queries that retrieve data.
Join us and order MediatR, a popular open-source library in the .NET ecosystem, to simplify the implementation of the Mediator pattern. MediatR serves as a mediator between different parts of an application, enabling loose coupling and promoting better organization of code.
With the MediatR library in .NET Core or .NET 6, developers can order and leverage the powerful features provided by these frameworks to streamline their development process.
Improved Scalability and Performance
One significant advantage of implementing CQRS with MediatR is the potential for improved scalability and performance. By separating read and write operations in an orderly manner, developers can optimize data access patterns based on specific requirements.
For example, when handling write operations in order, developers can focus on ensuring consistency and reliability without being burdened by complex querying logic. On the other hand, read operations can be optimized for performance by utilizing dedicated query models tailored to specific use cases.
By decoupling these concerns through CQRS and leveraging MediatR's capabilities, applications can efficiently scale to handle increased workloads while maintaining optimal performance levels. In other words, by implementing CQRS and utilizing MediatR, applications can effectively manage and process a higher volume of orders without sacrificing performance.
Seamless Integration with ASP.NET Core or .NET 6
ASP.NET Core and .NET 6 offer excellent support for integrating CQRS and MediatR into your application. These frameworks provide built-in features that streamline the adoption of these patterns without excessive boilerplate code. By leveraging ASP.NET Core and .NET 6, you can easily order and manage the execution of commands and queries in your application.
To implement CQRS using MediatR in ASP.NET Core or .NET 6, follow these steps in order.
- To begin, in order to install the required packages, add the MediatR and
DependencyInjection
fromMediatR.Extensions.Microsoft
NuGet packages to your project. - Define commands and queries in order to separate the classes for commands (write operations) and queries (read operations). These classes should encapsulate the necessary data and logic for each operation.
- Implement handlers: For each command or query, create corresponding handler classes that implement the
appropriate MediatR interfaces (
IRequestHandler
orIRequestHandler
). - To ensure that MediatR can resolve the appropriate handlers when needed, register the required services using the built-in dependency injection container in your application's startup configuration in the specified order.
- Dispatch requests: Finally, in your application's code, use MediatR's mediator to dispatch commands or queries to their respective handlers.
By following these steps, you can harness the power of CQRS and MediatR within your ASP.NET Core or .NET 6 application effortlessly.
Installing Required NuGet Packages for MediatR
To start using MediatR in your project, you will need to install the "MediatR" NuGet package. This package is
essential as it provides all the necessary functionality to work effectively with MediatR. However, depending on
your specific requirements and project setup, you may also need to install additional packages such as
DependencyInjection
from MediatR.Extensions.Microsoft
for seamless integration with dependency injection.
Installing the required NuGet packages is a straightforward process that can be done through Visual Studio or the .NET Core CLI. Once installed, you'll have access to a range of powerful features and capabilities offered by MediatR.
Installing the "MediatR" Package
To get started, follow these steps to install the "MediatR" package:
- Open your project in Visual Studio.
- Right-click on the project name in the Solution Explorer and select "Manage NuGet Packages."
- In the NuGet Package Manager window, search for "MediatR."
- Select the latest version of "MediatR" from the search results.
- Click on the "Install" button next to the selected package.
- Review any dependencies that will be installed alongside MediatR and click "OK" to proceed.
- Once installation is complete, you're ready to start utilizing MediatR in your project.
Additional Packages for Dependency Injection Integration
If you're using a dependency injection container like Microsoft's built-in service provider or any other
compatible container, installing the DependencyInjection
from MediatR.Extensions.Microsoft
package is crucial for
seamless integration.
Follow these steps to install this additional package:
- Open your project in Visual Studio.
- Right-click on the project name in Solution Explorer and select "Manage NuGet Packages."
- Search for
DependencyInjection
fromMediatR.Extensions.Microsoft
. - Choose the latest version of the package from the search results.
- Click on the "Install" button to initiate the installation process.
- Carefully review any dependencies that will be installed and click "OK" to proceed.
By installing this package, you'll gain access to extension methods that simplify the registration of MediatR-related services in your dependency injection container, allowing for a smooth integration experience.
With both the MediatR
and DependencyInjection
from MediatR.Extensions.Microsoft
packages installed, you're now
equipped with all the necessary tools to leverage MediatR effectively within your project. These packages
provide a wide range of features, including request/response handling, query processing, notification
broadcasting, and more.
Setting up ASP.NET Core API with MediatR for CQRS Pattern
To leverage the power of the Command Query Responsibility Segregation (CQRS) pattern in your ASP.NET Core API project, you can integrate MediatR, a popular mediator library that simplifies communication between different components. NET Core API with MediatR to implement the CQRS pattern effectively.
Configuring Your ASP.NET Core API Project
The first step is to configure your ASP.NET Core API project to use the necessary services provided by the
Mediator package through dependency injection (DI). By adding the required dependencies in your Startup.cs
file,
you can ensure that MediatR is available throughout your application. This allows you to easily handle commands,
queries, and notifications using its features.
Once you have added the necessary dependencies, you need to register handlers and behaviors for commands, queries, and notifications within your DI container configuration. This step involves mapping each handler or behavior class with its corresponding interface or base class. By doing so, MediatR can identify and execute the appropriate logic based on incoming requests.
Properly Configuring Controllers for Mediator Pipeline
To receive requests through the mediator pipeline in your controllers, you must ensure they are properly
configured. Start by injecting an instance of IMediator
into your controller's constructor.
This provides access to all the capabilities offered by MediatR within your controller actions.
With access to IMediator, you can now send commands or queries from your controllers using methods like Send()
or
Publish()
. These methods allow you to pass requests along with any required data directly to their respective
handlers or behaviors registered earlier.
By utilizing this setup, you create a clear separation between command and query operations within your API project. Commands modify data while queries retrieve data without making any changes. This segregation helps maintain a more structured and scalable codebase.
Integrating Entity Framework Core with MediatR
If you are using Entity Framework Core as your data access technology, you can seamlessly integrate it with MediatR. By leveraging the power of both frameworks, you can achieve a highly efficient and maintainable application architecture.
To integrate Entity Framework Core with MediatR, create separate handler classes for each command or query that requires database operations. Within these handlers, utilize the appropriate methods provided by Entity Framework Core to interact with your database. This ensures that the CQRS pattern is followed consistently throughout your application.
Handling Product Commands in MediatR
Creating, updating, and deleting products are common CRUD operations in any application dealing with product management. In MediatR, a popular library for implementing the mediator pattern in .NET applications, handling these product commands is straightforward and efficient.
Command Classes for Product Operations
To handle different product-related operations, such as creating, updating, or deleting a product, we need to define specific command classes. These classes encapsulate the necessary data required to perform the desired operation. For example:
public class CreateProductCommand : IRequest
{
public string Name { get; set; }
public decimal Price {get; set; }
// Additional properties as needed
}
public class UpdateProductCommand : IRequest
{
public int Id { get; set; }
public string Name { get; set; }
public decimal Price { get; set; }
// Additional properties as needed
}
public class DeleteProductCommand : IRequest
{
public int Id { get; set; }
}
By defining separate command classes for each operation, we can easily pass the required parameters and maintain clarity in our codebase.
Implementing Command Handlers
Once we have defined our command classes, we need to implement corresponding command handlers that contain the logic to execute these operations. A command handler is responsible for processing a specific type of command and performing the necessary actions.
For instance, let's consider a PlaceOrderHandler
that handles the creation of a new product:
public class CreateProductHandler : IRequestHandler
{
private readonly IProductRepository _productRepository;
public CreateProductHandler(IProductRepository productRepository)
{
_productRepository = productRepository;
}
public async Task Handle(CreateProductCommand request, CancellationToken cancellationToken)
{
var newProduct = new Product { Name = request.Name, Price = request.Price};
await _productRepository.Add(newProduct);
return newProduct.Id;
}
}
In this example, the CreateProductHandler
receives a CreateProductCommand
and performs the necessary steps to
create a new product in the repository. The handler interacts with the repository, saving the newly created
product and returning its generated identifier.
Leveraging MediatR for Command Routing
MediatR simplifies command routing by automatically directing commands to their corresponding handlers. Instead of manually wiring up each command with its respective handler, we can rely on MediatR's built-in capabilities.
To send a command using MediatR, we can inject an instance of IMediator
into our desired class and call its
Send method:
public class ProductService
{
private readonly IMediator _mediator;
public ProductService(IMediator mediator)
{
_mediator = mediator;
}
public async Task CreateProduct(string name, decimal price)
{
var command = new CreateProductCommand { Name = name, Price = price };
return await _mediator.Send(command);
}
}
By utilizing MediatR's powerful routing mechanism, we can focus on defining our commands and handlers without worrying about manual wiring or complex routing logic.
Triggering and Testing Notifications in MediatR
Defining Notification Classes
In MediatR, notifications are used to represent events or messages that need to be broadcasted within your application. These notification classes serve as a way to communicate important information to other parts of the system. By defining these classes, you can ensure that relevant handlers are notified whenever a specific event occurs.
For example, let's say you have an e-commerce application and you want to send a notification whenever a new
order is placed. You can create a NewOrderNotification
class that contains all the necessary information about
the order, such as the customer details, items purchased, and total amount.
Sending Notifications with MediatR
Once you have defined your notification classes, you can use MediatR's Publish method to send out those notifications. This method will notify all registered handlers that are interested in those specific events. It acts as a central hub for broadcasting notifications throughout your application.
To publish a notification using MediatR, simply call the Publish method and pass in an instance of the desired notification class. MediatR will then take care of notifying all relevant handlers asynchronously.
Writing Unit Tests for Notifications
Testing is an essential part of software development, and it is no different when working with notifications in MediatR. To ensure that notifications are triggered correctly and handled by the appropriate handlers, it is crucial to write unit tests.
When writing unit tests for notifications, there are a few key aspects to consider:
- Arrange: Set up the necessary test data and dependencies.
- Act: Trigger the event or message by publishing the corresponding notification.
- Assert: Verify that the expected actions have been taken by checking if the appropriate handlers were invoked or if any side effects occurred.
By following this arrange-act-assert pattern, you can effectively test whether your notifications are being triggered and handled as expected.
For example, in our e-commerce application scenario, you can write a unit test to ensure that the
NewOrderNotification
is properly handled by the appropriate handlers. You can mock the necessary dependencies,
publish the notification, and then assert that the expected actions were taken, such as sending an email
confirmation to the customer or updating inventory levels.
Updating Datastore and Optimizing Data Transfer Objects with MediatR
Using MediatR to Handle Datastore Updates
Such as a database, using a reliable and efficient tool is crucial. This is where MediatR comes into play. MediatR is a powerful library that allows you to handle commands responsible for updating data in your datastore seamlessly.
With MediatR, you can define commands that encapsulate the logic required to update your data store. These commands can be easily executed by invoking their corresponding handlers. By utilizing this pattern, you can keep your code organized and maintain separation of concerns.
For example, let's say you have a FakeDataStore class that simulates a database. You want to update the
information of a specific user in this datastore. Instead of directly manipulating the FakeDataStore instance
throughout your codebase, you can define an UpdateUserCommand
> that contains all the necessary information for
updating the user's details.
public class UpdateUserCommand : IRequest
{
public int UserId { get; set; }
public string NewName { get; set; }
// Additional properties for other updated fields
}
Using MediatR, you can create an UpdateUserCommandHandler
that handles this command and performs the necessary
operations on the FakeDataStore. This way, any updates related to users are centralized within this handler.
Optimizing Data Transfer Objects with AutoMapper
Optimizing data transfer objects (DTOs) is essential. DTOs act as intermediaries between your domain models and
external systems, such as APIs or UI components. To simplify the mapping process between domain models and DTOs,
leveraging tools like AutoMapper
can significantly streamline development.
AutoMapper
provides an easy-to-use mechanism for defining mappings between objects with similar properties. By
configuring AutoMapper
, you can eliminate the need for manual mapping code and reduce potential errors that may
arise during this process.
For instance, let's say you have a User
domain model with various properties such as Id
, Name
, and Email
. To
create a corresponding DTO
, you can define a separate class called UserDto
with identical properties.
public class UserDto
{
public int Id { get; set; }
public string Name { get; set; }
public string Email { get; set; }
}
By utilizing AutoMapper
, you can easily map between the User model and the UserDto
without writing repetitive
mapping code manually. This not only saves time but also reduces the chances of introducing bugs due to human
error.
Validating Input Data with MediatR Validators
Ensuring proper validation of input data is crucial before performing any updates in your application. MediatR provides integration with validators, allowing you to validate command inputs effortlessly.
By utilizing MediatR validators, you can define validation rules for your commands using attributes or custom validator classes. These validators automatically execute before invoking the corresponding command handler, ensuring that all required data is valid and meets specified criteria.
For example, let's consider our previous UpdateUserCommand
. We want to ensure that the provided user ID is
positive and that the new name has a minimum length of three characters.
Querying Products by ID and All Products using MediatR
Implement query classes for retrieving products based on their unique IDs or fetching all available products.
When working with a complex system that involves retrieving data, it's important to have a structured approach. In the case of querying products, we can implement query classes that allow us to fetch specific products based on their unique IDs or retrieve all available products.
By creating a GetProductsQuery
class, we can define the necessary parameters needed to retrieve specific
products. This query class can include properties such as ProductId
or any other relevant identifiers. With this
implementation, we have a clear and defined way to request specific product information.
On the other hand, if we want to fetch all available products without any filters, we can create another query
class called GetAllProductsQuery
. This class doesn't require any additional parameters since its purpose is to
return all existing product data.
Develop query handlers that encapsulate the logic required to fetch the requested data from your datastore.
Once our query classes are in place, it's time to develop query handlers that encapsulate the logic needed to fetch the requested data from our datastore. These handlers act as intermediaries between our application and the underlying database or storage system.
For example, when handling the GetProductsQuery
, we can create a corresponding handler called
GetProductsQueryHandler
. Within this handler, we write code that retrieves product information based on the
provided ID
. We can interact with our datastore using SQL queries or any other suitable method depending on our
technology stack.
Similarly, when dealing with the GetAllProductsQuery
, we create a separate handler named
GetAllProductsQueryHandler
. This handler is responsible for fetching all available product data from our
datastore without any filtering conditions.
Utilize MediatR's Send method to dispatch queries and retrieve results efficiently.
To streamline our querying process and make it more efficient, we can leverage MediatR's Send method. MediatR is a powerful library that helps us implement the mediator pattern in our applications.
By using the Send method, we can dispatch our query objects to their corresponding handlers. MediatR takes care of finding the appropriate handler based on the query type and executing it. This simplifies our codebase by removing the need for manual handler selection and invocation.
When querying products, we can utilize MediatR's Send method to dispatch both the GetProductsQuery
and
GetAllProductsQuery
. The results are then returned back to us, allowing us to process and present the retrieved
data efficiently.
Receiving Responses and Working with Requests in MediatR
Handling Responses from MediatR
After executing requests through MediatR, it's essential to handle the responses returned by command or query handlers. These responses provide valuable information that can be further processed or used to present feedback to users. By effectively managing these responses, you can enhance the overall functionality and user experience of your application.
When working with MediatR, a request/response pattern is leveraged to facilitate communication between different components of your application. This pattern allows for seamless interaction between various parts of the system, enabling efficient handling of both read operations (queries) and write operations (commands).
Extracting Relevant Information
One of the key aspects when dealing with responses in MediatR is extracting relevant information for further processing. Depending on the nature of your application request, this information could be critical for making decisions or taking appropriate actions.
For example, let's consider an application where users can submit queries to retrieve specific data. When a query is executed through MediatR, the response returned may contain relevant details such as the requested data or any errors encountered during execution. By extracting this information from the response, you can process it accordingly and provide accurate feedback to the user.
To extract relevant information from a response in MediatR:
- Access the necessary properties or methods within the response object.
- Retrieve any required data or error messages.
- Store or process this extracted information as per your application's requirements.
- Present meaningful feedback to users based on the extracted details.
Leveraging Request/Response Patterns
MediatR offers powerful request/response patterns that streamline communication between different components within your application architecture. This approach promotes loose coupling and separation of concerns by adhering to principles like Query Responsibility Segregation (CQRS).
By leveraging these patterns provided by MediatR:
- You can clearly define distinct commands and queries within your application, enhancing code organization and maintainability.
- Command handlers can focus on executing write operations without worrying about returning values, as they are primarily concerned with modifying the system's state.
- Query handlers can concentrate on retrieving data and generating responses, ensuring read operations are efficiently handled.
This separation of responsibilities allows for better scalability, testability, and overall system performance. It also simplifies future enhancements or modifications to your application by isolating specific functionalities within their respective handlers.
Registering and Testing Behavior with MediatR
Behaviors play a crucial role in the MediatR library, allowing developers to register specific actions that intercept requests before they reach their respective handlers. By incorporating behaviors into your Dependency Injection (DI)> container configuration, you gain the ability to implement cross-cutting concerns such as logging, validation, or authorization seamlessly.
Register Behaviors within Your DI Container Configuration
When working with MediatR, registering behaviors is an essential step in customizing request processing. By configuring your DI container to include these behaviors, you can intercept requests and perform additional actions before they are handled by their corresponding handlers.
To register a behavior, follow these steps:
- Define a class that implements the
IPipelineBehavior
interface. - Implement the Handle method within your behavior class to add custom logic.
- Configure your DI container to include the behavior using the appropriate registration method.
For example, suppose you want to log every incoming request before it reaches its handler. You can create a logging behavior that writes relevant information about each request to a log file or another logging mechanism of your choice.
Implement Cross-Cutting Concerns with Behaviors
One of the significant advantages of using behaviors in MediatR is their ability to address cross-cutting concerns effectively. These concerns are aspects of an application that cut across multiple modules or layers and require consistent handling throughout the system.
By leveraging behaviors, you can encapsulate common functionality like logging, validation, or authorization in a single place without scattering it across various handlers. This approach promotes code reuse and ensures that these concerns are consistently applied throughout your application.
Consider implementing validation as a behavior within MediatR. Instead of duplicating validation logic across individual handlers, define a behavior that validates incoming requests based on predefined rules or business requirements. This approach centralizes validation logic and simplifies maintenance efforts.
Write Unit Tests for Behavior Execution
To ensure that behaviors are correctly applied and executed during request processing, it is essential to write comprehensive unit tests. Testing behaviors helps validate their functionality, ensuring they perform as expected when intercepting requests.
When writing unit tests for behaviors, consider the following:
- Test that the behavior correctly intercepts requests and performs the desired actions.
- Verify that the behavior does not interfere with the normal execution of handlers.
- Validate any modifications made by the behavior to the request or response objects.
By thoroughly testing your behaviors, you can identify and resolve any issues early on, ensuring reliable and predictable request processing within your application.
Harnessing the Power of MediatR
In conclusion, MediatR is a powerful library that allows developers to implement the CQRS pattern in their ASP.NET Core or .NET 6 applications. By using MediatR, you can separate your application's concerns and achieve better maintainability and scalability.
Throughout this guide, we have explored various aspects of using MediatR. We started by understanding the purpose and benefits of MediatR, including its ability to decouple components and simplify communication between them. Then, we delved into the practical implementation of CQRS and MediatR in an ASP.NET Core or .NET 6 project.
We discussed how to install the required NuGet packages for MediatR and set up an ASP.NET Core API with MediatR for the CQRS pattern. We also covered topics such as handling product commands, triggering and testing notifications, updating datastores, optimizing data transfer objects (DTOs), querying products by ID or all products, receiving responses, working with requests, registering and testing behavior.
If you want to enhance your development process by adopting a clean architecture approach like CQRS and leverage the power of message-based communication between components within your ASP.NET Core or .NET 6 application, implementing MediatR is a wise choice. It will enable you to write cleaner code that is easier to test and maintain while improving overall performance.
Now it's time for you to put what you've learned into practice! Start harnessing the power of MediatR today and take your application development skills to new heights.
FAQs
Can I use MediatR in other frameworks besides ASP.NET Core or .NET 6?
MediatR is a versatile library that can be used in various frameworks and platforms, not limited to just ASP.NET Core or .NET 6. It is compatible with other versions of .NET Framework and even works with Xamarin applications.
Is MediatR suitable for small projects or only for large-scale applications?
MediatR can be beneficial for both small and large-scale projects. While it provides significant advantages in complex applications, its simplicity and decoupling capabilities make it equally useful in smaller projects.
Are there any performance considerations when using MediatR?
When properly implemented, the performance impact of MediatR is minimal. However, like any architectural choice, it's essential to consider factors such as message size, network latency, and overall system complexity to ensure optimal performance.
Can I combine MediatR with other design patterns?
Absolutely! MediatR can be combined with various design patterns such as Dependency Injection (DI), Event Sourcing, and Domain-Driven Design (DDD). This flexibility allows you to build robust architectures tailored to your specific needs.
Is there community support available for MediatR?
Yes, MediatR has a vibrant community of developers who actively contribute to its development and provide support through forums, GitHub repositories, and online communities. You can find additional resources and examples from the community to further enhance your understanding of MediatR.