Mastering CQRS: A Beginner's Guide to Command Query Responsibility Segregation
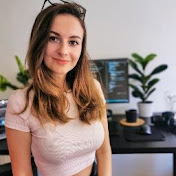
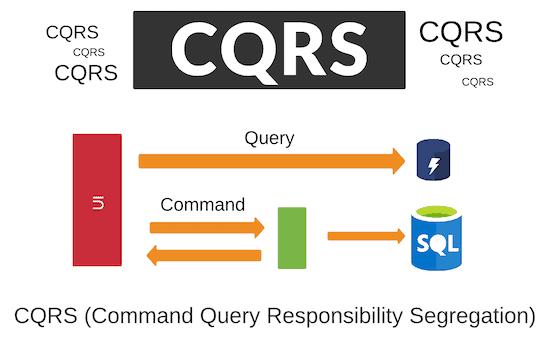
Command Query Responsibility Segregation (CQRS) is a design pattern that has gained popularity in recent years, particularly in the development of complex applications. The pattern involves separating the responsibility of handling commands and queries, which can lead to better scalability and performance.
At its core, CQRS involves creating separate handlers for commands and queries. The command handler is responsible for executing operations that change the state of the domain model, while the query handler is responsible for reading data from the database without modifying it. This separation allows developers to optimize read operations separately from write operations, leading to improved performance.
One of the key benefits of implementing Command Query Responsibility Segregation (CQRS) is achieving a clearer separation of concerns in code. By separating commands and queries into different handlers, developers can more easily maintain and extend their code over time. Additionally, transactions can be managed more efficiently with Command Query Responsibility Segregation (CQRS).
In order to implement CQRS effectively, it's important to understand how it works with domain models. Commands are used to modify the state of the domain model, while queries are used to retrieve data from it. By separating these two types of operations into different handlers, developers can achieve greater clarity and organization in their code.
What is CQRS? An Overview of Command Query Responsibility Segregation
The concept of Command Query Responsibility Segregation (CQRS) is an architectural pattern that separates the responsibilities of reading and writing data in a system. CQRS pattern involves splitting the application into two distinct parts: the command side, which handles write operations, and the query side, which handles read operations. By separating these concerns, CQRS can improve the performance, scalability, and maintainability of complex systems.
The command side of CQRS is responsible for handling write operations such as creating or updating data. It is designed to be optimized for high-performance writes and is typically implemented using a message-driven architecture. The query side, on the other hand, is responsible for handling read operations such as retrieving data from a database or cache. It is designed to be optimized for high-performance reads and can be implemented using various technologies like SQL databases or NoSQL databases.
The benefit of using Command Query Responsibility Segregation
One major benefit of using Command Query Responsibility Segregation (CQRS) is improved performance. By separating read and write operations into separate components, each component can be optimized for its specific task. This means the query component can be optimized for fast reads while the command component can be optimized for fast writes. Separating these concerns also makes it easier to scale each component independently based on its specific needs.
Another benefit of using Command Query Responsibility Segregation (CQRS) is improved scalability. Because each component can be scaled independently based on its specific needs, it's possible to scale only those components that need scaling without affecting others unnecessarily. This means that you don't have to over-provision resources just because one part of your system requires more resources than another.
Command Query Responsibility Segregation (CQRS) also improves maintainability by making it easier to reason about your codebase. Because each component has a well-defined responsibility, it's easier to understand what code should go where and how different parts of your system interact with each other. This makes it easier to make changes to your codebase without introducing unexpected bugs or breaking existing functionality.
Implementing Command Query Responsibility Segregation (CQRS) does require some additional complexity compared to traditional monolithic architectures. However, the benefits of improved performance, scalability, and maintainability make it a worthwhile tradeoff in many cases. Additionally, there are many tools and frameworks available that can help simplify the process of implementing CQRS.
Implementing CQRS Pattern: When, How, and Why?
When implementing Command Query Responsibility Segregation (CQRS), an architect must create separate read and write models, as well as separate write stores to handle the processing of write data. The read model is responsible for handling queries and returning results to clients. On the other hand, the write model handles commands that modify the state in response to user input.
One benefit of using CQRS is that it can help reduce contention between reads and writes. In a traditional architecture where reads and writes are handled by a single model or database table, contention can occur when multiple users try to access or modify the same data simultaneously. By separating reads from writes, CQRS can help alleviate this issue.
Another advantage of using Command Query Responsibility Segregation (CQRS) is that it allows for more flexible scaling options. Since read activity typically outweighs write activity in most applications, it may be possible to scale out read models independently from write models. This means that with CQRS you could potentially add more servers or instances to handle increased traffic on the read side without having to worry about scaling up your entire application.
Keep in mind CQRS is not a silver bullet for every performance problem!
When implementing CQRS, it's important to keep in mind that there is a trade-off between simplicity and flexibility. While CQRS can provide benefits in terms of performance and scalability, it also adds complexity to your application architecture. You will need to carefully consider whether the benefits of using CQRS outweigh the costs of implementation.
In addition, you should be aware that CQRS is not a silver bullet solution for all performance or scalability issues. Depending on your specific use case, other architectural patterns or techniques may be more appropriate than the CQRS pattern. It's important to thoroughly evaluate your options before deciding on a particular approach.
One example of where CQRS has been successfully implemented is in e-commerce applications with high traffic volume. By separating read and write activity, these applications are able to handle large numbers of concurrent users without experiencing contention or performance degradation.
Another example of the beneficial use of CQRS is in financial trading systems where low latency is critical for success. By separating reads from writes, these systems are able to achieve faster response times for queries while still maintaining consistency and reliability.
Benefits of CQRS Pattern in Software Development
Improved Performance
One of the most significant benefits of CQRS is that it separates read and write operations, allowing developers to optimize each operation independently. This means that developers can focus on improving the performance of either read or write operations without affecting the other.
For example, an e-commerce website may have thousands of users browsing products at any given time, but only a few hundred users making purchases. With CQRS, developers can optimize the read operations for browsing products while optimizing write operations for making purchases. This results in faster read and write operations, improving overall application performance.
Scalability
Another benefit of the CQRS pattern is scalability. Since CQRS allows developers to scale read and write operations independently, it makes it easier to handle high-traffic applications. This means the application can handle more users and requests without compromising performance.
For instance, imagine a social media platform with millions of active users who are posting updates every second. With CQRS, developers can scale up the read operation to handle more user requests while scaling down the write operation since fewer users will be posting updates.
Flexibility
CQRS pattern also provides flexibility in choosing different data stores for read and write operations. Developers can use different data stores optimized for each operation type; this makes it easier to choose the right data store for each operation type.
For instance, consider an online banking system where customers need fast access to their account balance while transactions require strong consistency guarantees. In the case of using the CQRS pattern, developers can use an in-memory cache for reading account balances while using a traditional relational database for transaction writes.
Simplified Codebase
CQRS pattern addresses the challenge of maintaining complex codebases by separating read and write operations into different codebases. This makes it easier to maintain and update each codebase independently, reducing the risk of introducing bugs or errors when updating the application.
For example, consider a blogging platform where users can create posts and view other users' posts. With CQRS, developers can separate read and write operations into different codebases. The read operation codebase can be optimized for browsing posts while the write operation codebase can be optimized for creating new posts.
Common CQRS Pattern Problems and Solutions
CQRS can cause problems like eventual consistency. This happens when the read model is updated slower than the write model, making them different. This can confuse users. To fix this, event sourcing can be used.
Event sourcing stores all changes as a sequence of events rather than just storing the current state of an object. With event sourcing, developers can replay events to reconstruct any previous state of an object accurately. This approach ensures that both read and write models are always consistent with each other.
While CQRS offers many benefits, it also comes with increased complexity and development time. Developers must carefully consider whether CQRS is appropriate for their project before implementing it. They should weigh the benefits against its drawbacks and determine whether it's worth investing additional time and resources into implementing it.
One way to mitigate these challenges is by using a framework or library that supports CQRS patterns. Many popular frameworks such as Axon Framework or EventStore provide built-in support for event sourcing and command query separation patterns.
Advanced Concepts and Techniques in CQRS
CQRS is an approach that can be particularly useful for more complex applications, where the separation of concerns between write and read operations becomes increasingly important. As applications grow in complexity, it's important to adopt practices that can help manage that complexity. CQRS can help by providing a clear separation between commands (writes) and queries (reads).
One advanced technique in CQRS is the use of materialized views. These are pre-calculated views of data that can be used to speed up read operations, particularly for complex queries. Materialized views can be challenging to implement, but they can provide significant performance benefits for read-heavy applications.
Materialized Views: An Advanced Technique
Materialized views are a powerful tool for optimizing read performance in CQRS systems. They work by pre-calculating the results of complex queries and storing them as tables in a database. When a query is executed against the materialized view, the database simply returns the pre-calculated results instead of executing the query from scratch.
This approach has several advantages over traditional query execution:
- Faster response times: Because materialized views are pre-calculated, they can return results much faster than traditional queries.
- Reduced load on servers: By offloading query processing to materialized views, servers are freed up to handle other tasks.
- Improved scalability: Materialized views allow for highly optimized query processing, which makes it easier to scale up as demand grows.
However, there are also some challenges associated with using materialized views:
- Increased storage requirements: Because materialized views store pre-calculated results, they require additional storage space.
- Increased maintenance overhead: Materialized views need to be kept up-to-date with changes to underlying data sources.
- Increased complexity: Implementing materialized views requires additional development effort and may introduce new points of failure into a system.
Trade-offs Between Write and Read Performance
When adopting CQRS, it's important to carefully consider the trade-offs between write and read performance. In some cases, it may make sense to prioritize one over the other.
For example, in a system where writes are infrequent but reads are frequent, it may be beneficial to optimize for read performance by using materialized views. On the other hand, in a system where writes are frequent and latency is critical, it may be necessary to prioritize write performance over read performance.
Ultimately, the decision of how to balance writing and read performance will depend on the specific requirements of each application.
CQRS and Event Sourcing: A Powerful Combination
CQRS (Command Query Responsibility Segregation) and event sourcing are two patterns that complement each other well, providing a powerful combination for building scalable and resilient systems. In this section, we will explore how these two patterns work together to create a robust and reliable system.
The benefits of using cqrs with Event Sourcing!
The event sourcing pattern records all changes to an app as a list of events. This helps keep track of all actions taken and rebuild past states by replaying events. It's great for keeping accurate records and debugging problems.
Multiple Services Can Subscribe To The Same Event Log
When using event sourcing and CQRS together, multiple services can subscribe to the same event log and update their own data stores independently. This approach allows for eventual consistency while avoiding merge conflicts between different services' data stores.
For instance, consider a case where multiple services need access to customer information but require different views of this information based on their specific needs. By subscribing to the same event log and updating their own data stores independently, each service can create its own view without interfering with other services' views or causing merge conflicts.
Ensuring Business Logic Is Kept Separate From Data Store Concerns
Another advantage of using CQRS and event sourcing together is that it ensures business logic is kept separate from data store concerns. By separating commands and queries, the business logic can be focused on implementing the desired behavior of the system without being concerned with how this behavior is stored or retrieved.
This separation allows for easier maintenance of the codebase since changes to the data storage layer will not affect the business logic layer. It also makes it easier to test and reason about individual components of the system.
Performance Benefits
Event sourcing and CQRS can provide significant performance benefits for certain scenarios. For instance, by using event sourcing, it becomes possible to optimize write operations for performance since all changes are appended to an event log instead of updating a traditional database.
Similarly, using CQRS, read operations can be optimized for scalability since each service can create its own view of the data based on its specific needs. This approach allows for horizontal scaling of read operations across multiple services while keeping write operations centralized in a single location.
When to Use CQRS Pattern in Your Software Projects
As software projects grow in complexity, it becomes increasingly important to use design patterns that can handle the demands of complex business logic. One such pattern is Command Query Responsibility Segregation (CQRS), which separates read and write operations into different models. In this section, we will explore when to use CQRS in your software projects.
Handling Complex Business Logic
CQRS is useful when you have complicated business tasks that need separate read-and-write models. For instance, an online store needs to check product availability, calculate shipping costs, and update inventory levels when you place an order. A single model that handles both reading and writing can become too complicated. CQRS helps by using different models for reading and writing, making each task easier.
Improved Performance
Another benefit of using CQRS is improved performance. By separating read and write operations into different models, you can optimize each model for its specific task. For example, you might use a NoSQL database for fast writes and a relational database for complex queries.
This separation also allows for independent scaling of read and write components. This means that if your application needs more read capacity than write capacity (or vice versa), you can scale each component independently without affecting the other.
Reducing Complexity with Large Datasets
Working with large datasets can be challenging, especially when it comes to data retrieval and manipulation. Using CQRS can help to reduce this complexity by separating read and write operations into different models.
For example, consider a social media platform with millions of users who generate thousands of posts per minute. Retrieving all of this data at once would be prohibitively complex. However, by using CQRS, you can separate the read and write operations into different models that are optimized for their specific tasks.
When to Use CQRS
So when should you use CQRS in your software projects? Here are some situations where it can be particularly useful:
- When you need to handle complex business logic that requires different read and write models.
- When you need to improve performance by allowing for optimized read and write operations.
- When you need to scale your application horizontally, it allows for independent scaling of read and write components.
- When working with large datasets, using CQRS can help to reduce the complexity of data retrieval and manipulation.
Why CQRS is a Game-Changer for Modern Software Development
CQRS is a pattern that can change how software is developed. It separates commands from queries to make applications easier to maintain and improve over time. CQRS helps improve performance because developers can optimize each side of the application independently. This means queries can be done faster without affecting the performance of commands. CQRS also simplifies domain modeling by focusing on modeling business logic in terms of commands and events.