Reflection in C#: A Comprehensive guide with examples and benefits of using it
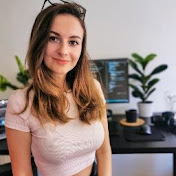
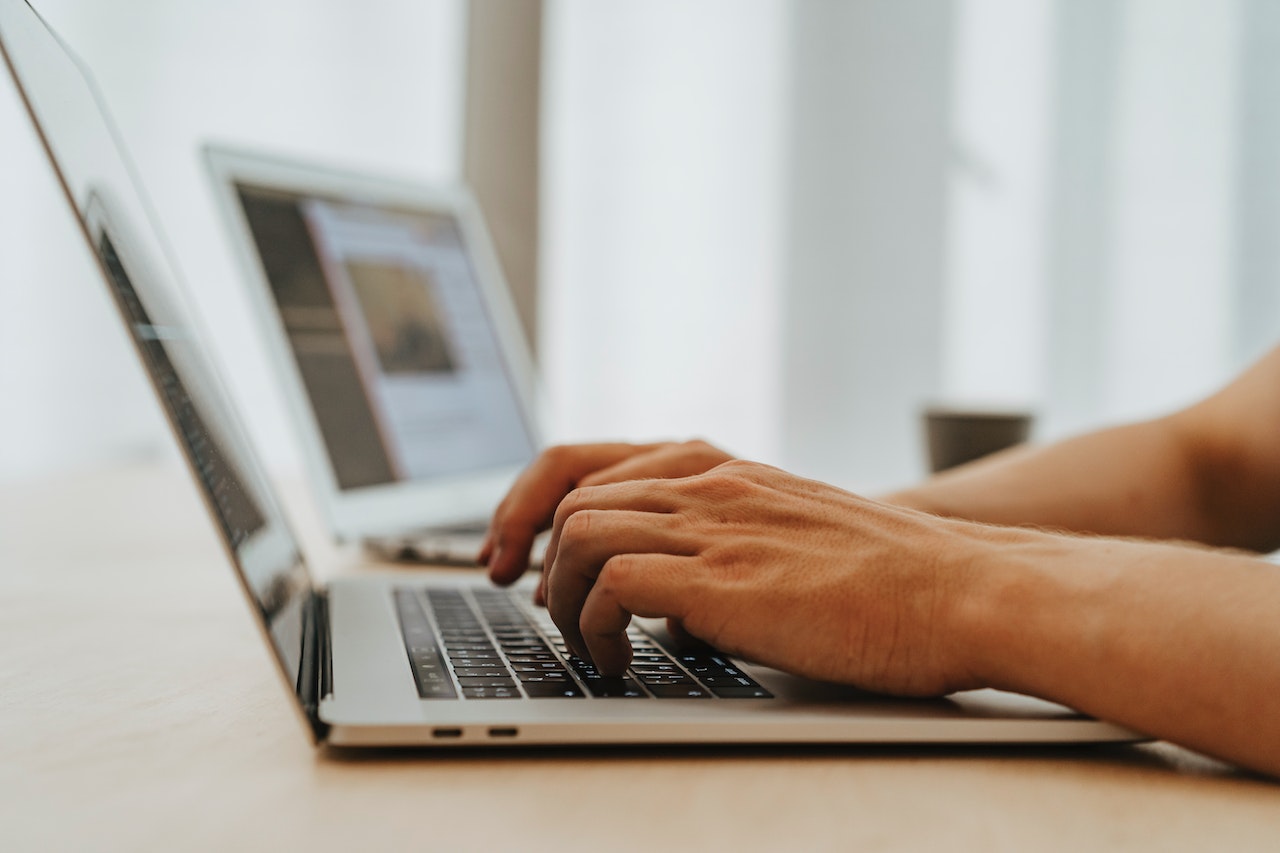
Have you ever wondered how some advanced C# applications are able to perform tasks that seem almost magical? How they can dynamically load assemblies, access private members, and even generate code on the fly? The secret lies in a powerful feature called reflection.
Reflection is like having X-ray vision for your code. It allows you to examine and manipulate the structure of types at runtime. Think of it as peeking behind the scenes and gaining access to information that is normally hidden from view.
With reflection in C#, you can dynamically load assemblies, inspect metadata, and create instances of types. This opens up a world of possibilities for building flexible and extensible applications. Need to invoke a method dynamically? No problem! Reflection has got you covered.
But why should you care about reflection? Well, it plays a crucial role in frameworks like ASP.NET MVC and Entity Framework. These frameworks rely on reflection to perform various tasks behind the scenes, such as mapping database tables to classes or routing HTTP requests to controllers.
Understanding reflection is essential for advanced C# programming. It empowers you to build applications that are not bound by static constraints but can adapt and evolve at runtime.
Understanding the concept of reflection in C#
C# reflection is a powerful feature that allows you to obtain information about types (classes, interfaces, structs) at runtime. It opens up a whole new world of possibilities by enabling you to analyze type metadata such as properties, methods, events, constructors, and more.
Retrieving type information dynamically with reflection in C#
One of the key benefits of reflection is the ability to retrieve attributes associated with types or members. Attributes provide additional information about an object or entity and can be used for various purposes such as validation, serialization, or custom behavior. With reflection, you can easily access these attributes at runtime and make decisions based on their values.
For example, let's say you have a Student class with attributes like "Name," "Age," and "Grade." By using reflection, you can programmatically examine these attributes and perform actions accordingly. You could create a function that checks if a student is eligible for a scholarship based on their grade or retrieve the default values for each attribute.
Dynamic invocation and accessing private members with reflection in C#
Reflection also enables the dynamic invocation of methods. This means that instead of hard-coding method calls in your code, you can use reflection to invoke methods dynamically based on certain conditions or user input. This flexibility allows your program to adapt and respond to different scenarios at runtime.
Furthermore, reflection provides access to private members that are not directly accessible through normal means. Private members are typically hidden from external code but may contain valuable information or functionality. By leveraging reflection, you can bypass these restrictions and gain access to private fields, properties, or methods when necessary.
Harnessing the power of reflection effectively
To leverage the power of reflection effectively in your C# projects, it's important to understand how it works
internally. The Reflection namespace in C# provides classes like Type, MethodInfo, PropertyInfo
, etc., which
allow you to interact with type metadata programmatically.
By utilizing these classes, you can explore the structure of an object or entity at runtime. You can retrieve information about its properties, methods, events, constructors, and more. This knowledge empowers you to build dynamic and flexible applications that adapt to changing requirements.
For instance, imagine you have a Customer class with various properties like "Name," "Address," and "PhoneNumber." With reflection, you could create a function that dynamically generates a form based on the properties of the Customer class. This form would automatically adapt if new properties are added or existing ones are modified.
Benefits of using reflection in C#
Flexibility at its finest
Reflection in C# offers a level of flexibility that can greatly enhance your code. It allows you to work with unknown or dynamically loaded types, opening up a world of possibilities. With reflection, you can write code that adapts to different scenarios without the need for explicit knowledge of the types involved.
Imagine a situation where you have a plugin system in your application. Using reflection, you can dynamically load and interact with these plugins, even if they were not known at compile-time. This means that as new plugins are added or removed, your code can seamlessly adapt to these changes without requiring manual intervention.
Dynamic object creation made easy
One of the powerful features provided by reflection is the ability to dynamically create objects based on user input or configuration files. This is particularly useful when dealing with scenarios where the structure or type of objects needs to be determined at runtime.
Let's say you have an application that allows users to define custom data structures through a graphical interface. Reflection enables you to take those definitions and create corresponding objects on-the-fly, without needing to predefine each possible structure in advance. This gives your users the freedom to define their own data models and empowers them with greater control over their applications.
Building adaptable frameworks
Reflection's capability to discover type information at runtime opens up opportunities for building generic frameworks that can adapt to different types. By leveraging this feature, you can create highly flexible and extensible systems that cater to various scenarios.
Consider a scenario where you are developing a plugin framework for an application. With reflection, your framework can automatically discover and integrate new plugins without requiring modifications to the core codebase. This makes it easier for developers to extend your application's functionality by simply dropping in new plugins, reducing development time and effort.
Simplifying serialization/deserialization
Serialization and deserialization tasks often involve analyzing object structures and mapping them to a specific format. Reflection simplifies this process by automatically analyzing object structures without requiring explicit coding effort.
Let's say you have a complex object hierarchy that needs to be serialized into JSON or XML. With reflection, you can traverse the object graph and extract the necessary information without manually defining each property or field to be serialized. This saves you from tediously writing mapping code and makes the serialization process more efficient.
Empowering libraries and tools
Reflection plays a crucial role in empowering various libraries and tools, such as dependency injection containers and ORM frameworks. These tools rely on reflection to discover types, resolve dependencies, and perform other essential tasks.
For example, a dependency injection container uses reflection to scan your codebase for classes with specific attributes or interfaces, allowing it to automatically wire up dependencies at runtime. Similarly, an ORM framework utilizes reflection to map database tables to corresponding objects and perform CRUD operations seamlessly.
Limitations of using reflection in C#
Slower performance compared to direct access
One of the main drawbacks of using reflection in C# is its slower performance compared to direct access. Reflection involves additional overhead due to runtime analysis and lookup operations. When accessing members through reflection, the code needs to analyze and inspect the type at runtime, which can result in a significant performance hit. This extra processing time can be especially noticeable when working with large or complex codebases.
Security vulnerabilities with incorrect usage
Another limitation of using reflection is the potential for security vulnerabilities if it is not used correctly. Reflection allows access to private members and methods that are not intended for public use. If sensitive members are accessed or modified unintentionally, it can lead to security breaches or unexpected behavior in the application. Therefore, it is crucial to exercise caution and ensure that proper access controls are in place when utilizing reflection.
Violation of encapsulation principles
Reflection provides features like invoking private members, but their use should be judiciously considered as they may violate encapsulation principles. Encapsulation aims to hide internal implementation details and expose only necessary interfaces. By bypassing these restrictions through reflection, the code becomes more susceptible to breaking encapsulation and compromising the integrity of the system. Careful consideration should be given before resorting to invoking private members using reflection.
Breakage during refactoring
When using reflection-based functionality, one must be aware that refactoring code might break such functionality if there are changes in the structure of types. Since reflection relies on analyzing types at runtime, any modifications made during refactoring may render existing reflective code obsolete or non-functional. It is important to thoroughly test reflective components after any refactoring process to ensure their continued compatibility.
Code complexity and maintainability challenges
Reflection should be used sparingly as it has the potential to make code harder to understand and maintain over time. The dynamic nature of reflection makes it difficult to follow the flow of execution, as it bypasses compile-time checks and exposes code to potential errors that would otherwise be caught during compilation. Reflection-based code tends to be more verbose and less readable than its direct access counterparts. Therefore, it is recommended to limit the use of reflection to situations where it is absolutely necessary and ensure that proper documentation accompanies reflective code for easier understanding and maintenance.
Exploring System.Object.GetType() and System.Type.GetType()
The System.Object.GetType()
method and the System.Type.GetType()
method are essential tools in C# for obtaining
information about types at runtime using reflection. These methods allow you to navigate through complex type
hierarchies and perform dynamic operations. Let's dive into the details of each method and explore how they can
be used effectively.
Understanding System.Object.GetType()
The first method we'll discuss is System.Object.GetType()
. This method
returns the runtime type of an object.
In
other words, it tells you the actual type of an object at runtime. This is particularly useful when dealing
with
polymorphic behavior, where a variable can hold objects of different types.
For example, let's say you have a base class called Animal and two derived classes called Dog and Cat. You create an instance of the Dog class and assign it to a variable of type Animal. If you call the GetType() method on that variable, it will return the type as Dog, even though the variable is declared as type Animal.
This allows you to perform operations specific to that particular derived class, based on its actual runtime type. You can check if an object is of a certain type, compare types, or even dynamically invoke methods based on their types.
Exploring System.Type.GetType()
Next up is the static method called System.Type.GetType()
. This method
retrieves the type object for a given
type
name or assembly-qualified name. Unlike the previous method we discussed, this one is not called on an
instance
but rather on the Type class itself.
To use this method, you provide either just the name of a known type or its fully qualified assembly name. It then returns a Type object representing that particular type. This can be useful when you don't have an instance of an object but still need to obtain its type information.
For example, if you have a string variable containing the name of a type, you can pass that string to the GetType(string typeName) method. It will return the Type object representing that type, allowing you to perform various operations on it.
Leveraging Type Information and Type Class
Both System.Object.GetType()
and System.Type.GetType()
are fundamental in obtaining type information at
runtime.
By understanding how to use these methods effectively, you can unlock the power of reflection in C#.
Here are some practical examples of what you can do with these methods:
- Check if an object is of a certain type:
if (myObject.GetType() == typeof(MyType)) { // Perform specific operations for MyType }
- Get the name of a type:
Console.WriteLine(myObject.GetType().Name);
- Invoke a method dynamically based on its type:
MethodInfo method = myObject.GetType().GetMethod("MyMethod"); if (method != null) { method.Invoke(myObject, null); }
- Explore properties and fields of a type:
PropertyInfo[] properties = myObject.GetType().GetProperties(); foreach (PropertyInfo property in properties) { Console.WriteLine(property.Name); FieldInfo[] fields = myObject.GetType(). }
Utilizing reflection in C# to access properties, methods, and variables
Reflection is a powerful feature in C# that allows you to retrieve information about properties, methods, fields (variables), events, and more defined in a type. It provides a way to inspect and manipulate objects at runtime dynamically. Let's dive deeper into how reflection can be used to access public properties, methods, and variables within a type.
Retrieving property values with reflection
One of the key benefits of reflection is the ability to dynamically read or modify property values using PropertyInfo objects obtained through reflection. With these objects, you can access various attributes of the property such as its name, data type, whether it is readable or writable, and more.
For example, let's say we have a class called Person with public properties like Name, Age, and Address. Using reflection techniques, we can obtain PropertyInfo objects for these properties and then retrieve their values at runtime. This allows us to access property values without knowing their names beforehand.
Type personType = typeof(Person);
PropertyInfo[] properties = personType.GetProperties();
foreach (PropertyInfo property in properties)
{
Console.WriteLine($"Property Name: {property.Name}");
Console.WriteLine($"Property Value: {property.GetValue(person)}");
}
In the above code snippet, we obtain an array of PropertyInfo
objects
using the GetProperties()
method from
the
Type class. We then iterate over each property and print its name and value using the Name and GetValue()
methods provided by PropertyInfo
.
Invoking methods dynamically
MethodInfo objects obtained via reflection enable dynamic invocation of methods with different parameters at runtime. This means that you can call methods on an object without knowing them beforehand or having compile-time knowledge about them.
Let's consider a scenario where we have a class called Calculator
with
various public methods like Add,
Subtract
,
and so on. Using reflection, we can obtain MethodInfo objects for these methods and then invoke them
dynamically
based on user input.
Type calculatorType = typeof(Calculator);
MethodInfo[] methods = calculatorType.GetMethods();
foreach (MethodInfo method in methods)
{
if (method.Name == userInput)
{
method.Invoke(calculator, null);
break;
}
}
In the above example, we obtain an array of MethodInfo
objects using the
GetMethods()
method from the Type
class.
We iterate over each method and check if its name matches the user input. If there is a match, we invoke the
method dynamically using the Invoke() method provided by MethodInfo
.
Accessing variables with reflection
FieldInfo objects provide access to public or private variables within a type using reflection techniques. This allows you to examine and modify variable values dynamically at runtime.
Suppose we have a class called Car with public variables like Model, Year, and so on. By utilizing
reflection, we
can obtain FieldInfo
objects for these variables and then access their
values programmatically.
Type carType = typeof(Car);
FieldInfo[] fields = carType.GetFields(BindingFlags.Public |
BindingFlags.Instance);
foreach (FieldInfo field in fields)
{
Console.WriteLine($"Variable Name: {field.Name}");
Console.WriteLine($"Variable Value: {field.GetValue(car)}");
}
Real-time applications of reflection in C#
Extensive use in frameworks like ASP.NET MVC for model binding and action invocations based on route data In the world of web development, frameworks like ASP.NET MVC rely heavily on reflection to provide powerful features such as model binding and action invocations based on route data. Reflection allows these frameworks to dynamically analyze and manipulate objects at runtime, enabling them to automatically bind incoming request data to the appropriate model classes. For example, let's say you have a form submission in your web application that sends data to a controller action. The framework uses reflection to inspect the properties of the target model class and map the incoming form fields to those properties. This automatic mapping saves developers from writing repetitive code for every form submission. Reflection also plays a crucial role in invoking actions based on route data. When a user requests a specific URL, the routing mechanism maps it to an appropriate controller action using reflection. The framework analyzes the available actions, their parameters, and their attributes at runtime through reflection, allowing it to dynamically invoke the correct action method.
Utilized by ORM frameworks like Entity Framework for dynamic mapping with database entities
Object-Relational Mapping (ORM) frameworks like Entity Framework simplify database interactions by providing an abstraction layer between the application code and the underlying database. These frameworks utilize reflection extensively to map database entities with corresponding classes dynamically at runtime. With Entity Framework, developers can define entity classes that represent database tables. Reflection is then used by the framework to analyze these entity classes' properties and generate SQL queries accordingly. This dynamic mapping eliminates the need for manual SQL query construction or explicit configuration mappings between classes and tables. By leveraging reflection, ORM frameworks like Entity Framework offer flexibility and convenience when working with databases. Developers can focus more on defining business logic rather than dealing with low-level database operations.
Dependency injection containers rely on reflection for automatic dependency discovery
Dependency injection (DI) is a popular design pattern that promotes loose coupling and modular code. DI containers, such as Microsoft's built-in Dependency Injection framework in ASP.NET Core, rely on reflection to automatically discover dependencies and create instances without explicit configuration. When using a DI container, developers define interfaces and their corresponding implementations. The container then uses reflection to scan the application's assemblies for these interfaces and their implementations. By analyzing the types at runtime, the container can automatically instantiate dependencies when needed. This automatic dependency discovery saves developers from manually configuring each dependency explicitly. It also enhances maintainability as new implementations can be added without modifying existing configuration code. Reflection empowers DI containers to dynamically adapt to changes in the application's structure, making it easier to manage complex projects.
Serialization libraries like JSON.NET employ reflection for automatic object analysis during serialization/deserialization processes. Serialization is the process of converting objects into a format suitable for storage or transmission, while deserialization is the reverse process of recreating objects from serialized data. Reflection plays a vital role in serialization libraries like JSON.NET by allowing them to analyze object structures automatically during these processes. When serializing an object using JSON.NET, reflection is used to examine its properties and fields dynamically. This analysis enables the library to extract the necessary data from the object and generate a corresponding JSON representation accurately. During deserialization, JSON.NET utilizes reflection to create instances of target classes based on the provided JSON data.
Implementing reflection in C# for dynamic code execution
Loading assemblies dynamically at runtime.
Reflection plays a crucial role. One of the key features of reflection is the ability to load assemblies
dynamically at runtime. This allows you to incorporate external functionality into your application without
having to statically reference them during compilation. To load assemblies dynamically, you can use the
Assembly.Load()
or Assembly.LoadFrom()
methods. These methods enable you to specify the assembly's
location
and load it into memory at runtime. By doing so, you gain access to all types and members defined within
that
assembly.
Creating instances of types dynamically
Once you have loaded an assembly using reflection, you can leverage its power to create instances of types
dynamically. The Activator.CreateInstance()
method comes in handy here.
It allows you to instantiate
objects
without knowing their specific type at compile time. By providing the name of the type as a string
parameter,
Activator.CreateInstance()
creates an instance of that type on-the-fly.
This flexibility opens up
possibilities for building highly adaptable systems where new types can be introduced without requiring
changes
in your codebase.
Invoking methods dynamically
Reflection not only enables the creation of objects but also allows you to invoke methods dynamically. This
is
particularly useful when dealing with scenarios where method names or parameters are determined at runtime.
With
reflection, you can obtain a MethodInfo
object representing a particular
method and then invoke it using
appropriate parameters using the Invoke()
method. This capability
empowers developers to build flexible
systems capable of adapting and executing different logic based on changing conditions.
Achieving dynamic behavior at runtime
By leveraging reflection's capabilities for loading assemblies, creating instances of types, and invoking methods dynamically, developers can achieve dynamic behavior in their applications at runtime. This means that instead of relying solely on statically defined code, applications become more adaptable and able to respond to changes on-the-fly. For example, you can build plugins or extensions that are loaded dynamically at runtime, allowing your application to gain new functionality without requiring a recompilation. This flexibility is especially valuable in scenarios where you want to provide extensibility or allow users to customize the behavior of your software.
Creating dynamic proxies for cross-cutting concerns
Another powerful use case for reflection is the creation of dynamic proxies. Proxies act as intermediaries between clients and target objects, allowing you to intercept method calls and inject additional behavior. This technique is commonly used for implementing cross-cutting concerns such as logging, caching, or security checks. With reflection, you can generate proxy objects dynamically by creating a class that implements the same interfaces as the target object. By intercepting method invocations using reflection, you can add custom logic before and after the actual method execution. This approach provides a way to modularize and separate cross-cutting concerns from core business logic.
Enhancing code flexibility with reflection
Reflection in C# is a powerful feature that enables software developers to build more flexible and adaptable code. By leveraging reflection, you can write generic algorithms that dynamically adapt to different types without needing prior knowledge of those types. This ability opens up a world of possibilities for creating highly extensible and reusable code.
Discovering and utilizing custom attributes
One of the key benefits of using reflection is the ability to discover and utilize custom attributes attached to types or members. Custom attributes provide a way to attach additional metadata or behavior to your code elements. With reflection, you can programmatically access these attributes at runtime and use them to implement extensible behaviors. Imagine you have a project where you want to implement plugins or extensions that can be discovered automatically at runtime without the need for recompiling the main application. Reflection allows you to achieve this by scanning assemblies and finding types or methods marked with specific custom attributes. You can then load these assemblies dynamically, instantiate the required types, and invoke their methods as needed. This approach provides a great deal of flexibility in extending your application's functionality without modifying its core codebase.
Building frameworks with user-defined types
Reflection also empowers developers to build frameworks that support user-defined types. Instead of relying on specific hard-coded dependencies, you can design your framework in such a way that it leverages reflection to work with any compatible type provided by the user. For example, let's say you're developing a framework for handling data serialization. By using reflection, your framework can analyze the structure of any given type at runtime and generate serialization/deserialization logic accordingly. This allows users of your framework to serialize/deserialize their own custom classes without having to modify or recompile your framework itself. Reflection enables frameworks to extend their functionality through configuration files or external modules. For instance, your framework could allow users to define additional behaviors for certain types by specifying them in a configuration file. At runtime, your framework can use reflection to discover and apply these behaviors based on the provided configuration. This approach provides a high level of flexibility and customization options for users without requiring them to modify the core framework code.
Decoupling dependencies on specific types
Another significant advantage of using reflection is the ability to decouple dependencies on specific types. Reflection allows you to work with types dynamically, reducing the need for compile-time knowledge of those types. Consider a scenario where you have a method that needs to invoke different static methods based on user input. By using reflection, you can determine the appropriate method to invoke at runtime by analyzing the available methods in a given type. This approach eliminates the need for conditional statements or switch cases based on known types, making your code more flexible and adaptable. Reflection also enables you to work with enumerable types without explicitly knowing their concrete implementations. You can use reflection to iterate over collections or query objects dynamically without relying on compile-time information about their structure or interfaces. This flexibility allows your code to handle various data structures seamlessly, regardless of their underlying implementation.
Overcoming performance challenges with reflection optimization techniques
Reflection is a powerful feature in C# that allows you to examine and manipulate code dynamically at runtime.
While it offers numerous benefits, such as increased flexibility and the ability to access properties,
methods,
and variables dynamically, it also comes with its own set of challenges. Understanding the concept of
reflection
helps developers harness its full potential. By utilizing System.Object.GetType()
and
System.Type.GetType()
,
you can retrieve information about an object's type, enabling you to perform various operations based on
this
knowledge. However, using reflection extensively can lead to performance issues due to its inherent
overhead.
The benefits of using reflection must be carefully weighed against these limitations. To overcome
performance
challenges associated with reflection, optimization techniques can be employed. Real-time applications of
reflection in C# often require dynamic code execution. By implementing reflection for such scenarios, you
can
achieve greater flexibility in your code. Enhancing code flexibility is one of the key advantages of
utilizing
reflection. It allows you to write generic algorithms that work with different types without explicitly
knowing
their details beforehand. In order to optimize your use of reflection, consider employing caching mechanisms
to
avoid repetitive lookups and reduce runtime overhead. This approach ensures that the expensive reflective
operations are performed only once and subsequent invocations benefit from cached results. Another technique
involves leveraging Emit or Expression Trees for generating dynamic methods at runtime instead of relying
solely
on traditional Reflection APIs. These approaches offer better performance by bypassing some of the costly
reflective operations. In conclusion, while reflection provides invaluable capabilities in C#, it is
essential
to be mindful of its impact on performance. By understanding its concepts thoroughly and employing
optimization
techniques like caching and dynamic method generation, you can overcome these challenges effectively while
still
benefiting from the power and flexibility offered by reflection. So why wait? Start exploring the world of
reflection in C# today!