C# Record vs Class: Key Differences and Best Use Cases
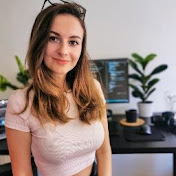
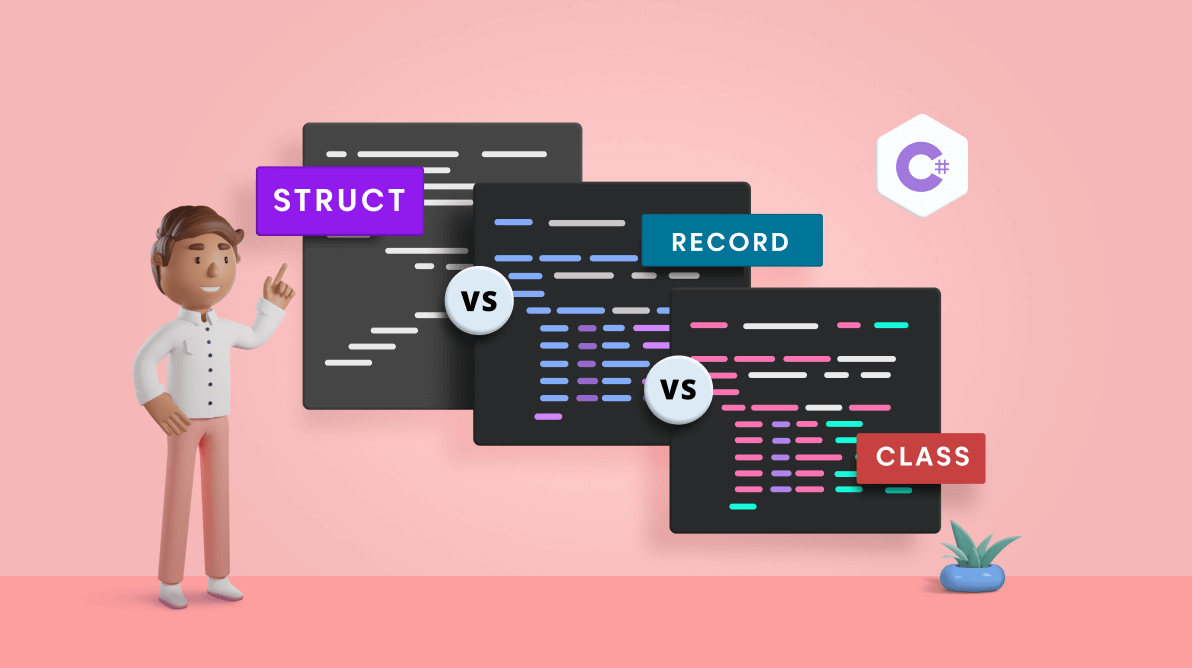
Are you a code-savvy developer looking to level up your C# game? Well, buckle up because we're about to dive into the intriguing world of C# record vs class. These two fundamental concepts in object-oriented programming hold the key to writing efficient and powerful code in C#.
But what sets them apart? That's precisely what we'll explore in this article - the crucial distinctions between C# record vs class. By understanding their differences, you'll be equipped with the knowledge needed to make informed decisions.
The Difference between C# Record vs Class
When comparing C# record vs class, they have distinct characteristics that set them apart. Understanding these differences is crucial for developers who want to make the right choice when designing their code.
Immutability and Mutability of C# records vs classes
One fundamental distinction between C# records vs classes lies in their mutability. While records are immutable by default, meaning their values cannot be changed once assigned, classes are mutable, allowing modifications to their properties.
Equality Semantics for C# records vs classes
Another key difference of C# records vs classes relates to equality semantics. Records provide value-based equality semantics, which means they compare the values of their properties for equality. On the other hand, classes use reference-based equality, comparing whether two objects refer to the same memory location.
Inheritance Support for C# records vs classes
Inheritance is an essential feature in object-oriented programming, but it varies between C# record vs class. While inheritance is not supported for records, it remains possible with classes. This distinction allows developers to create class hierarchies and derive new types from existing ones.
Understanding these differences helps developers choose between C# records vs classes type based on their specific requirements. Whether it's immutability versus mutability, value-based or reference-based equality semantics, or the need for inheritance support - each characteristic plays a role in determining which option is most suitable for a given scenario.
By considering these distinctions between C# records vs classes carefully, developers can leverage the strengths of both within their projects.
When to Use C# Record vs Class
There are a few points to consider when to use C# records vs classes.
Use a record when you need an immutable type with value semantics. Records are ideal for representing data
structures that are meant to be read-only and have value-based equality semantics. They provide a concise
syntax
for defining immutable objects and automatically generate useful methods like Equals, GetHashCode
, and
ToString
.
Choose a class when you require mutability or inheritance. Classes allow you to define mutable objects that can be modified throughout their lifetime. They also support inheritance, allowing you to create hierarchies of related types and leverage polymorphism.
Advantages of Using C# Records vs Classes
There are several advantages to using C# record vs class. Let's explore these benefits:
- The simplified syntax for defining immutable types: With C# records, you can easily define immutable types using a concise syntax. This simplifies the process of creating objects that cannot be modified once instantiated.
- Enhanced support for pattern matching and deconstruction: C# records provide improved support for pattern matching and deconstruction. This allows you to conveniently extract data from records and perform operations based on specific patterns or conditions.
- Improved performance due to structural equality checks: Unlike classes, C# records perform structural equality checks by default. This means that two record instances with the same values in their properties are considered equal. This feature enhances performance by eliminating the need for manual equality comparisons.
Using C# records vs classes can lead to more efficient and maintainable code. By leveraging simplified syntax, enhanced support for pattern matching and deconstruction, and improved performance through structural equality checks, developers can benefit from increased productivity and code clarity.
So, when working on projects that require immutability, pattern matching, or performance optimizations, consider utilizing C# records vs classes as they provide significant advantages over traditional classes.
Understanding the Key Features of C# Records
Having compared C# records vs classes, the former offers several key features that make them a powerful tool for developers. Let's explore these features in more detail:
- Automatic implementation of
GetHashCode()
andEquals()
methods based on property values:
Comparing C# records vs classes, with records, you don't have to manually override theGetHashCode()
andEquals()
methods. They are automatically implemented based on the property values of the record.
This simplifies the process of comparing and hashing records, saving developers time and effort. - Value-based equality comparisons through the == operator without overriding it explicitly:
In C#, when using records, you can compare instances using the == operator without explicitly overriding it.
The == operator performs value-based equality comparisons for record instances, making it easier to check if two records have identical property values. - Support for positional patterns in pattern-matching expressions:
C# records support positional patterns in pattern-matching expressions.
This allows developers to easily match specific patterns within record instances by their positions, enabling more flexible and concise code.
These features enhance productivity and simplify code maintenance when working with C# records vs classes. By automating common tasks like implementing equality comparisons and providing support for positional patterns, C# records enable developers to focus on building robust applications efficiently.
Comparing the Syntax and Behavior of C# Record vs Class
Syntax
Records in C# are defined using the "record" keyword, while classes are defined using the "class" keyword. However, classes can also include optional modifiers like "sealed" or "abstract."
Behavior
There is a fundamental difference between C# record vs class. Records have value semantics by default, meaning they are treated as data structures rather than reference types. On the other hand, classes have reference semantics unless explicitly overridden.
Here are some key differences to consider, comparing C# records vs classes:
- Records:
Are similar to immutable data structures.
Encourage public read-only fields for data members.
Provide structural equality out of the box.
Generate value-based equality methods automatically. - Classes:
Are more commonly used for complex behaviors and encapsulation.
Allow mutable state through properties and fields.
Rely on reference equality by default.
Require manual implementation of equality methods.
In terms of code examples, let's take a look at how these differences manifest:
// Record example
public record Person(string Name, int Age);
// Class example
public class PersonClass
{
public string Name { get; set; }
public int Age { get; set; }
}
In this simple comparison of C# record vs class representing a person's information, we can see that the record syntax is more concise and automatically generates useful methods like ToString(), GetHashCode(), and Equals() based on its members.
On the other hand, the class requires explicit implementation of these methods if needed. Since records have value semantics by default, two instances with identical values will be considered equal even if they occupy different memory locations.
Utilizing Record Types with Expressions in C#
Records can be a powerful tool when working with expressions in C#. By leveraging record types, you can simplify code generation and enable the creation of dynamic code at runtime. This opens up new possibilities for powerful code manipulation.
Here's how you can make the most out of record types and expressions:
- Simplify Code Generation: With record types, you can define data structures that are immutable by default. This means that once a record is created, its values cannot be changed. This immutability simplifies code generation as you don't need to worry about managing mutable state.
- Dynamic Code at Runtime: Expressions allow you to generate and execute code dynamically during runtime. By combining expressions with record types, you can create flexible and adaptable code that responds to different scenarios. This enables you to build more dynamic applications that can adjust their behavior based on changing conditions.
- Powerful Code Manipulation: The combination of record types and expressions gives you the ability to manipulate your code in powerful ways. You can dynamically create new instances of records, modify existing records, or even generate entirely new code based on certain conditions or inputs.
Best Practices for Using C# Records vs Classes
When to Use C# Records vs Classes
- Use records when immutability and value-based equality are desired.
- Prefer classes when mutability or inheritance is necessary.
Naming Conventions and Access Modifiers
- Follow naming conventions to maintain code readability.
- Make proper use of access modifiers for encapsulation.
Using C# records vs classes effectively requires understanding their purpose and applying best practices.
When immutability and value-based equality are desired, records offer a convenient solution. They allow you to create objects that are immutable by default, ensuring that once initialized, their values cannot be changed. This can be particularly useful when dealing with data transfer objects or representing entities where the values should remain constant. Records provide built-in value-based equality checks, making it easier to compare instances based on their content rather than reference.
On the other hand, if mutability or inheritance is necessary, classes are the way to go. Unlike records, classes can have mutable properties and methods that allow for changes after initialization. Furthermore, they support inheritance, enabling you to create hierarchies of related types.
To maintain code consistency and readability, it's important to adhere to naming conventions. Choose
meaningful
names for your record or class that accurately represent their purpose in the application. Make proper use
of
access modifiers such as public, private
, or protected
to control visibility and encapsulation.
By following these best practices when working with C# records vs classes:
- Utilize records for immutability and value-based equality requirements.
- Opt for classes when mutability or inheritance is needed.
- Apply appropriate naming conventions for clarity.
- Implement access modifiers effectively.
Case Studies: Real-World Applications of C# Record vs Class
Having compared C# records vs classes, both offer powerful tools for developers to model their domain and create complex object hierarchies. Let's explore some case studies that highlight the practical usage of records and classes in real-world applications.
Example scenarios showcasing the practical usage of records in domain modeling:
- When designing a system for managing employee data, using records allows us to define lightweight, immutable data structures that represent employees' information. This ensures type safety and simplifies the codebase.
- In a financial application, records can be used to model transactions, where each transaction is represented by a record instance containing relevant data members such as amount, date, and recipient.
Real-world applications demonstrating the benefits of using classes for complex object hierarchies:
- When building a game engine and comparing C# records vs classes, the latter is invaluable for creating complex object hierarchies. For example, we can use classes to define different types of game entities like characters, enemies, or items with varying behaviors and attributes.
- In large-scale enterprise systems, classes provide flexibility in representing business entities with intricate relationships. Class inheritance enables us to create specialized subclasses while reusing common functionality from base classes.
Explore case studies that highlight the appropriate use cases for both C# records vs classes:
- Domain Modeling of C# records vs classes:
Records excel at representing simple data structures with immutability.
Classes shine when dealing with complex hierarchical relationships between objects. - Data Structures of C# records vs classes:
Records are suitable for lightweight data containers without behavior.
Classes allow us to encapsulate behavior along with data in more elaborate structures.
By understanding these examples and considering the specific needs of your project, you can make informed decisions about the advantages of C# records vs classes.
C# Records vs classes: Making the Right Choice for Your Project
In conclusion, understanding the differences between C# records vs classes is crucial for making the right choice in your project. C# records provide several advantages over classes, such as immutability by default, enhanced syntax for equality comparison, and built-in support for value-based equality semantics.
When deciding whether to use C# records vs classes, consider the specific requirements of your project. Records are well-suited for scenarios where you need immutable data structures with value-based equality. Classes offer more flexibility and are suitable for situations that require mutable objects with behavior.
By utilizing C# records vs classes, you can take advantage of their key features like expression-bodied members and deconstructors. These features allow for concise code and improved readability.
It's important to consider performance considerations when choosing between C# records vs classes. While records may have some overhead due to their immutability characteristics, they can provide performance benefits in certain scenarios.
To make the most out of using C# records vs classes, follow best practices such as properly defining equality members, avoiding unnecessary inheritance hierarchies, and keeping your codebase clean and maintainable.
Real-world case studies demonstrate how distinguishing advantages of C# records vs classes have been successfully applied in various projects. By exploring these examples, you can gain insights into how others have leveraged these language features to solve specific problems.
In summary, understanding the strengths and weaknesses of C# records vs classes will empower you to make informed decisions about which approach is best suited for your project's needs. Embrace the power of C# records vs classes to write cleaner code with improved expressiveness while maintaining high-performance standards.